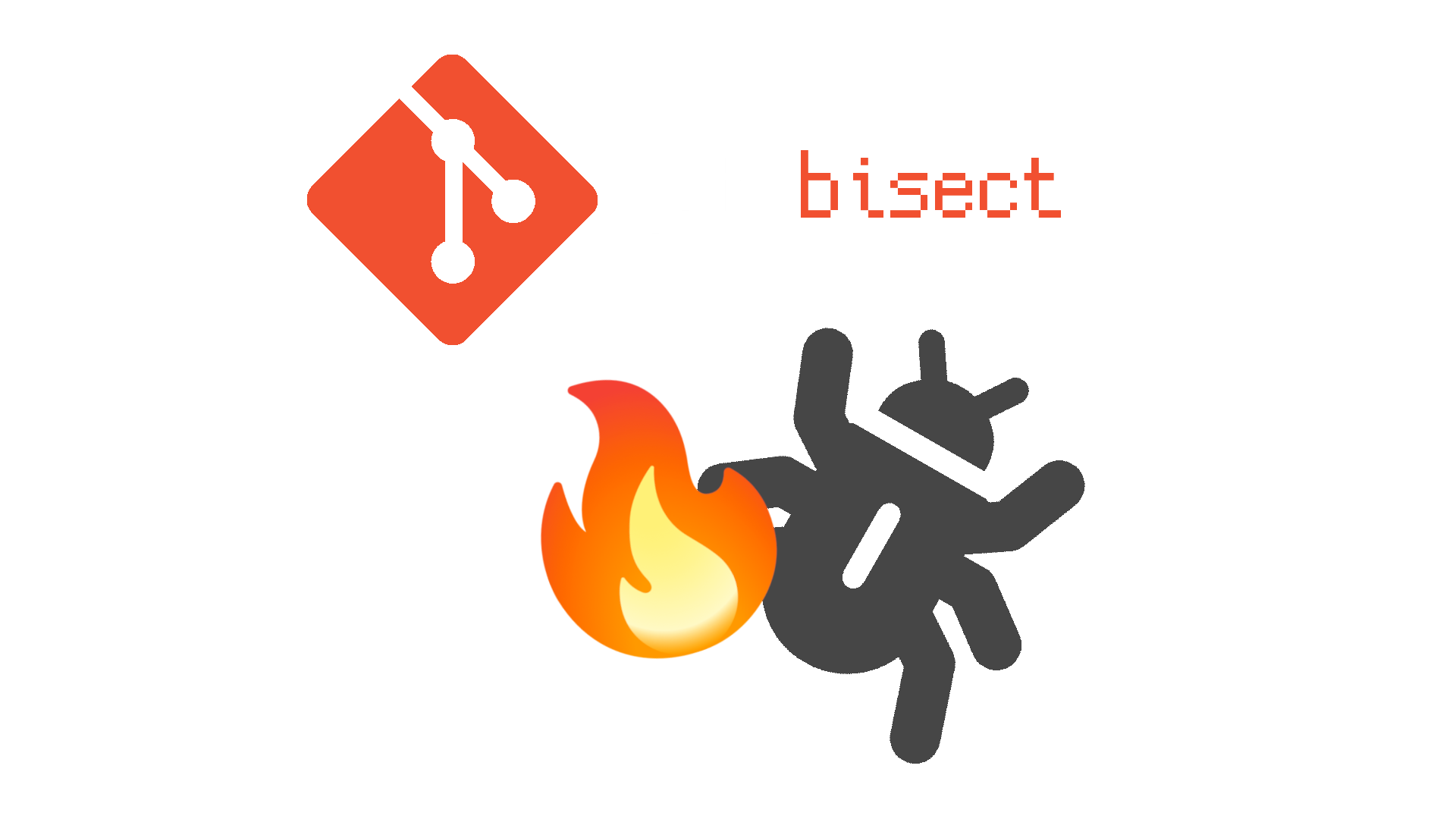
Tracking down bugs in your code — using git bisect
Finding the specific commit that introduced a bug in your code can be frustrating, especially in big projects with a lot of commits. Git bisecting is a method used to quickly find which commit is the culprit. Git bisect works by you specifying a so called 'bad' commit where you know the bug occurs and a commit where you know the bug doesn't occur. Afterwards git will binary search it's way to find the commit introducing the bug.
Suppose we've the following git history:
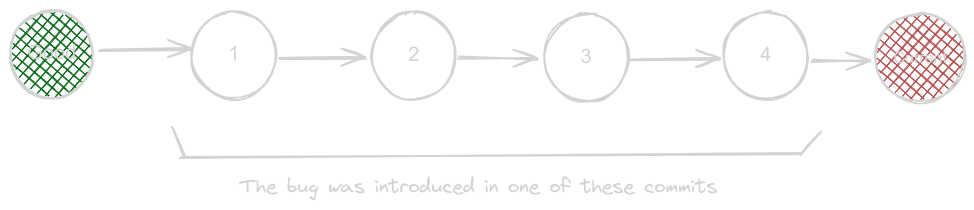
It could potentially contain many more commits between the known 'good' commit and the current one. Somewhere in the commits 1, 2, 3, 4 or the current one, a bug was introduced. One way to find the specific commit that introduced the bug, could be to check each commit starting from commit 1 then 2 then 3 ... and so on. This is known as a linear search, and would take very long if there are a lot of commits between the bad and the current.
Instead git bisect comes to the rescue. Git bisect performs a binary search, which is much faster. To use git bisect, you must tell git to start bisecting:
$ git bisect start
Afterwards we mark the 'bad' commit - any commit we know the bug occurs in. In this example
the current commit that we know is bad have the commit hash c26cf8a
, so
we mark the commit bad:
$ git bisect bad c26cf8a
After that we mark a previous commit that we know the bug doesn't occur in. In this
example it's the 'good' commit (se picture above), which has a commit hash of b34ec52
$ git bisect good b34ec52
Now git will automatically checkout a commit somewhere in between the good and bad commit. Your job is now to re-build your project and test if the bug occurs. If the bug doesn't occur you report it to git:
$ git bisect good
However if it does occur you mark it bad:
$ git bisect bad
You continue to do this until git has tracked down the first bad commit, ie. the commit that introduced the bug.